One of the most common requirements for any app is to have an authentication flow that includes onboarding at the same time. The flow looks like the following –
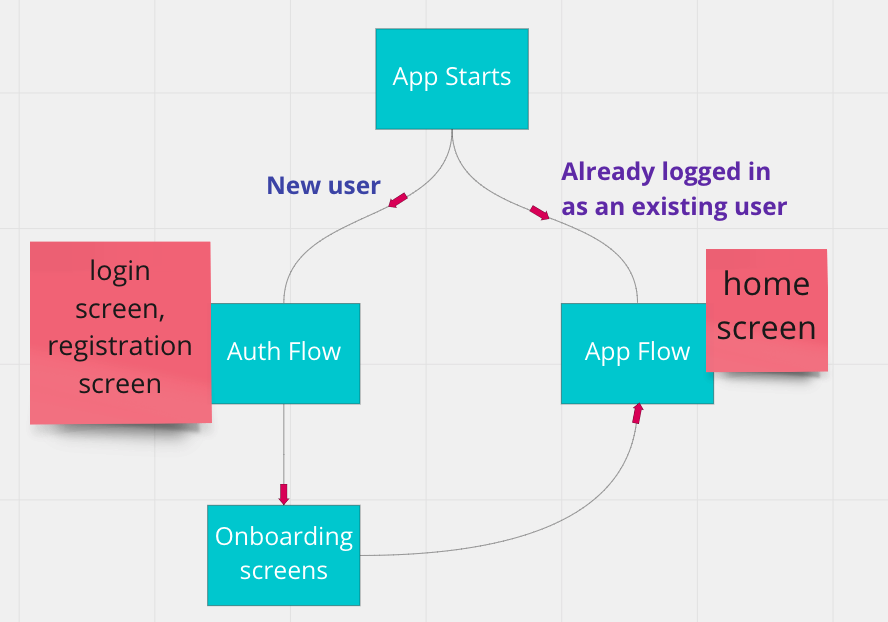
- When user opens the app, we first need to check if the user is a logged-in user in our system, depending on this condition they can go to two different flows;
- If the user has already been logged in previously, they will come to the app screens. for ex – home screen, profile screen etc.
- If the user is new or a logged-out user, they will go to the authentications screens, for ex – login page, signup page etc.
- For a completely new user, when they signup for the first time we also want to show them an onboarding screens where usually we would show the app’s main features/advantages or app’s how-to-guide. Upon completion of the onboarding screes they will continue to the app’s main screes. (logged-in flow)
Setting up the screens
We will create a few dummy screens to show the flow properly. We will have –
- Landing Screen
- Login Screen
- Signup Screen
- Onboarding Screen
- Home Screen
If we want to describe the screen flow, then it would look like the following –

Auth Context
To manage the user authentication flow, we will be using context. Let’s start by creating our AuthContext.

For the sake of the example, we will try to keep things simple. We create auth context and pass 2 values from our context provider to the whole app. The values are
isLoggedIn
– which is a boolean value that lets us know if our user is logged in or not.setIsLoggedIn
– setisLoggedIn
state to either true or false depending on the login flow.
Let us wrap the app with AuthProvider.
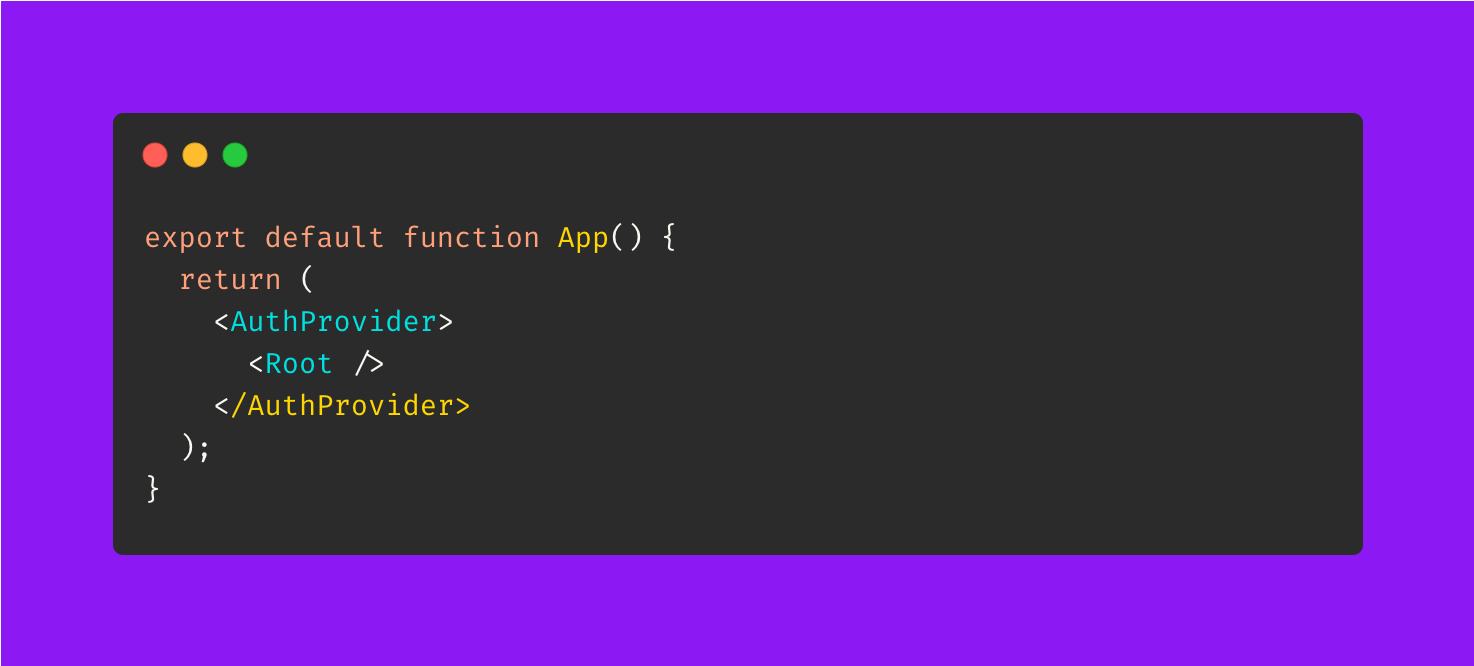
Root Component
With that setup done, we will now set up our screens. Our initial component is Root. Inside our Root, we will check if the user is logged in or not. Depending on the isLoggedIn value from our context we will redirect the user to proper flow.
Initially, our Root Component will look like this

Login
We will first set up the login flow. In our login screen.
- We will have a button called login and when pressing the button we will use
setIslogged
it to make ourisLoggedIn
state in our context totrue
. - When
isLoggedIn
becomes true, the user will automatically navigate toHomeScreen
. Which we already set up in ourRoot
. - We also use
AsyncStorage
to persist a dummy token value so that when users close the app we can use the persist value to know if they are already logged in or not.
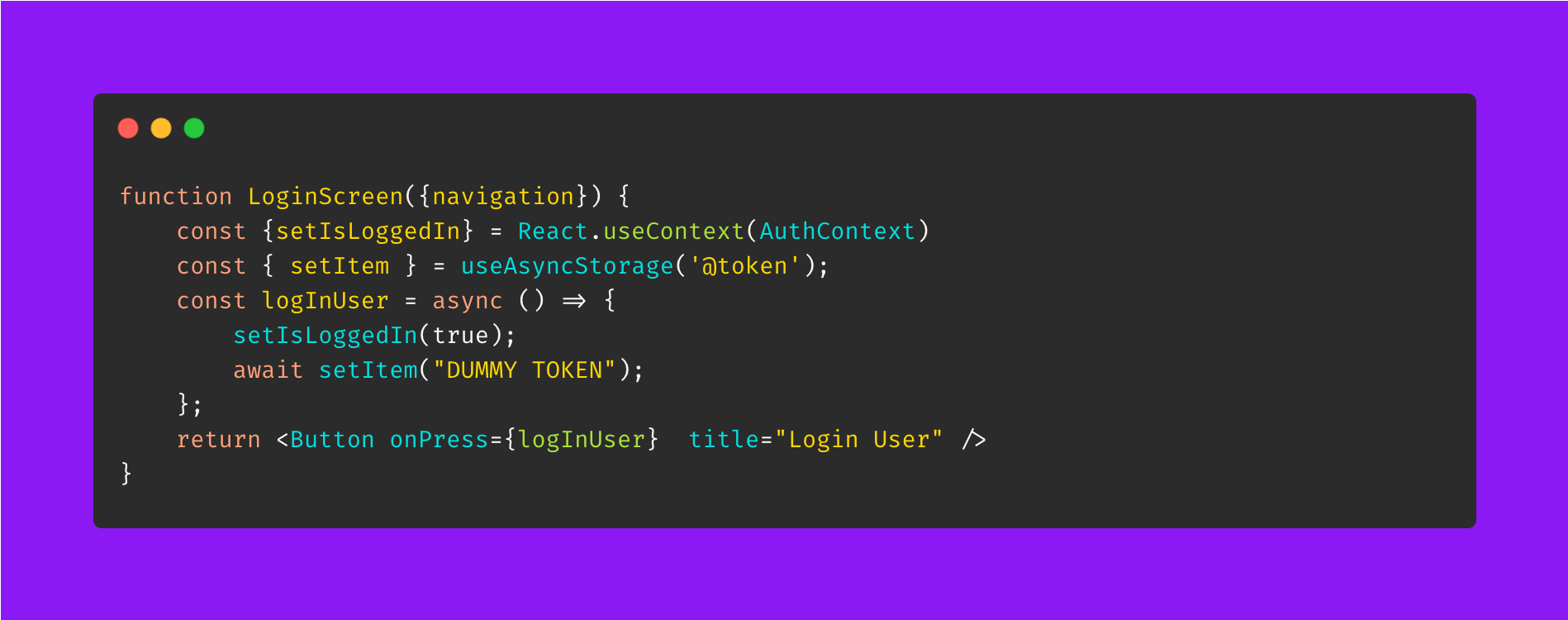
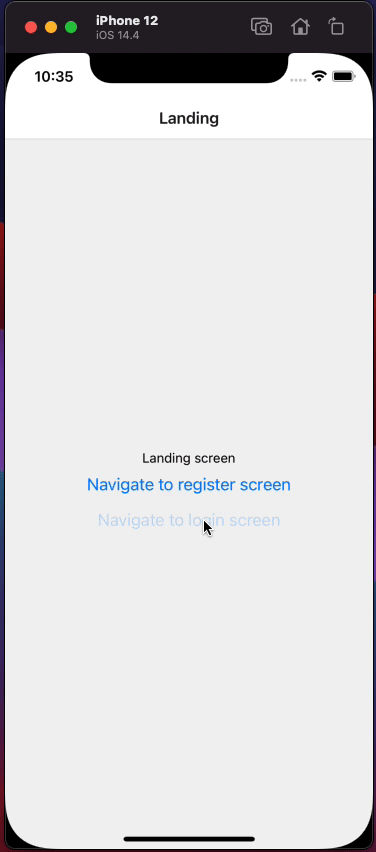
Signup and Onboarding
For new users, we want them to signup and go through the onboarding flow. Once they complete the onboarding flow, we want them to go to the main app.

- lets assume we have a button on signup screen, upon clicking the button the registration is successful and we navigate the user to the onboarding screen

- Onboarding screen is doing exactly the same as login screen. It is making the isLoggedIn value to true and save a dummy token in our async storage. When it sets the isLoggedIn to true, the user will automatically go to the main app.
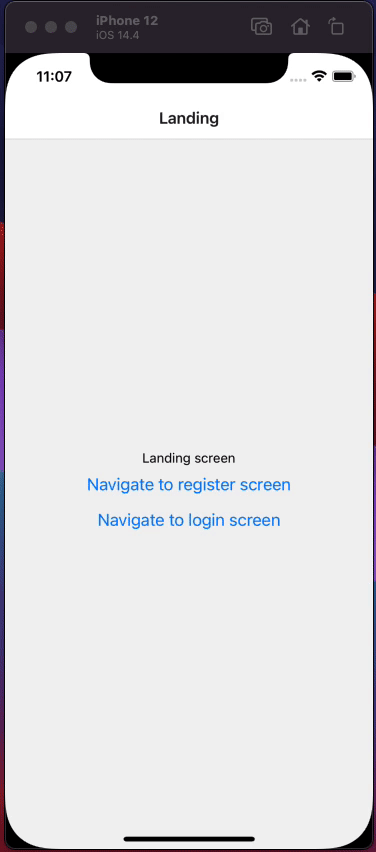
Home & Logout
On our home screen, we will have a logout button. If a user clicks the logout button we will do 2 things
- Make
isLoggedIn
false - Remove dummy token from our async storage
- When we make
isLoggedIn
to false, user will automatically go toLandingScreen
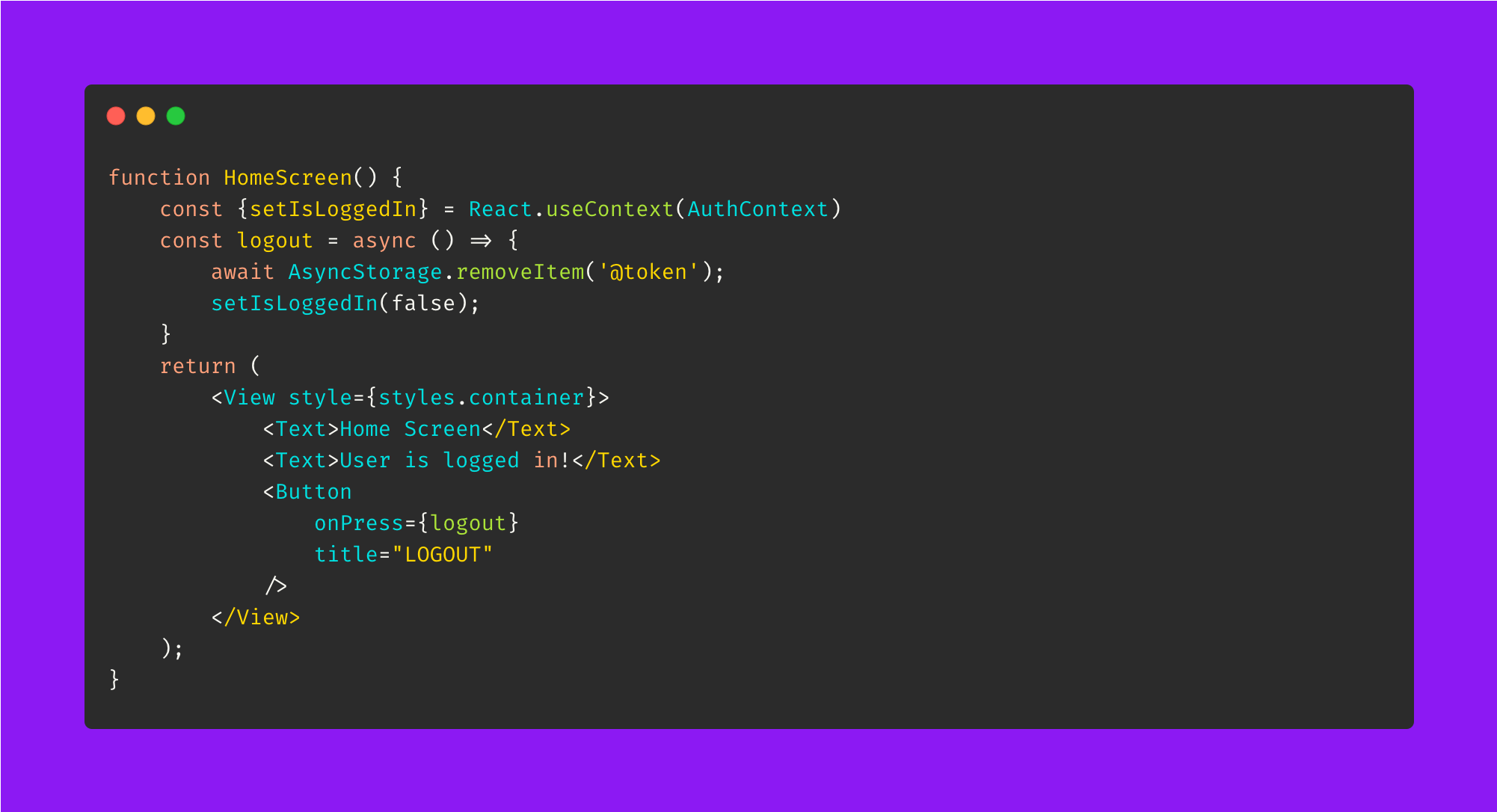
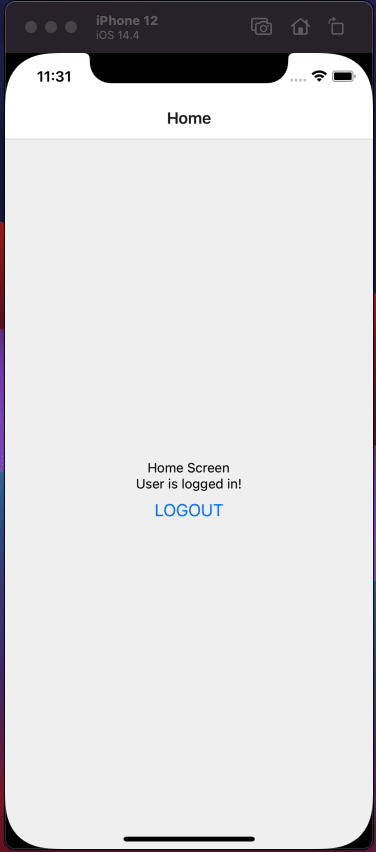
Check if user is logged in from AsyncStorage
Whenever the user logs in or signs up we were saving a dummy token in our to persist it. We wanted to persist the token because if users close the app and come back to our app sometime later we want to keep them in the same state. We will do this checking in our Root Component.

- When our component first loads, we try to get the token from async storage, if the token is found then we make the
isLoggedIn
value to true by callingsetIsLoggedIn(true)
- We also use a loader state, since getting the token is async, we will need to wait and show a loading indicator until the check is complete.
Now, let us see this in action, We will first login, close the app, and come back to the app again. Our user should still be on the home screen, not on the landing screen.

With that our full flow is complete! Here is the snack link if anyone wants to grab the code. Thanks!