We all know the common user experience of going through a terms & conditions page. User needs to scroll down to the bottom to enable the submit button and only after that, they can proceed. We will try to build a small demo of the same experience in this post.
First, let us see the end product to get a clear picture of what we are trying to achieve.
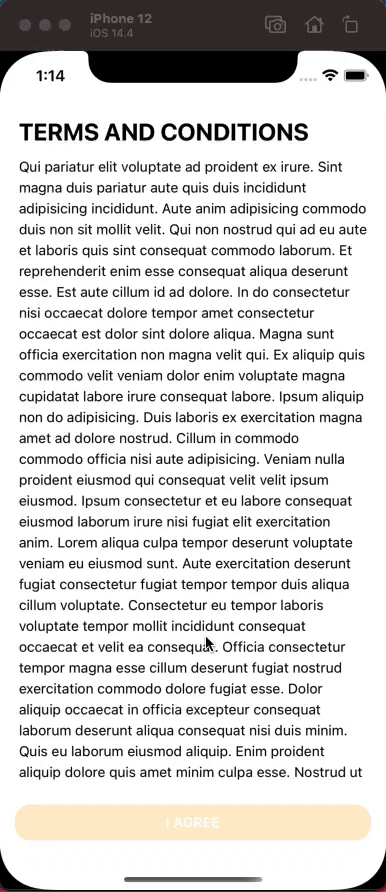
From the demo, we can point out a few key areas that need to be tackled to implement this behavior.
- First we need to detect if the user has reached to the bottom of the page, if they reach to a certain point at the bottom we will need to enable the disable button.
- We will also need to track the progress of the scroll. So that we can show the progress bar according to the scroll position to the user. User will have a clear idea of how long they have progressed.
- Finally to make the UX more friendly we can animate the button opacity when the button gets enabled.
Alright, let’s start with the implementation.
1. Detecting if user has reached to the bottom of the screen:
At the initial phase, our button is disabled. We can create a state to change the button enable/disable state, like so –
const [btnDisable, setBtnDisable] = React.useState(true)
To detect the user’s scroll position we will use the onScroll
props of our ScrollView
.
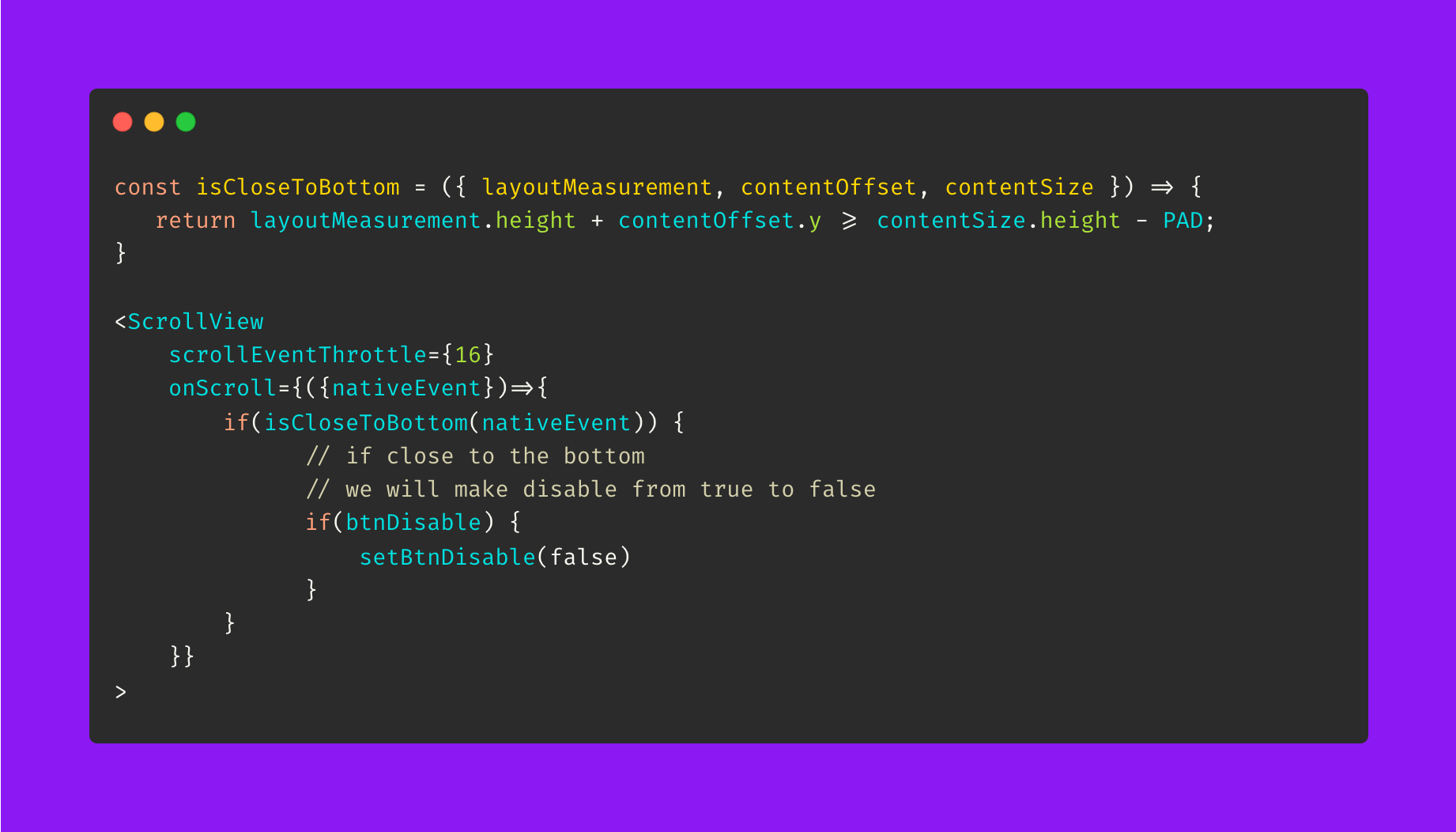
2. Enable the button with an opacity animation:
From the above code, we can see that we are enabling the button. We can add a simple opacity animation to create a nicer feeling. Initially, the opacity value is 0.2
. That’s why we see a pale orange value which lets the user know that the button is still inactive.
const fadeAnim = useRef(new Animated.Value(0.2)).current;
We can make the opacity value to 1 when we set the value of the btnDisble
state to false.
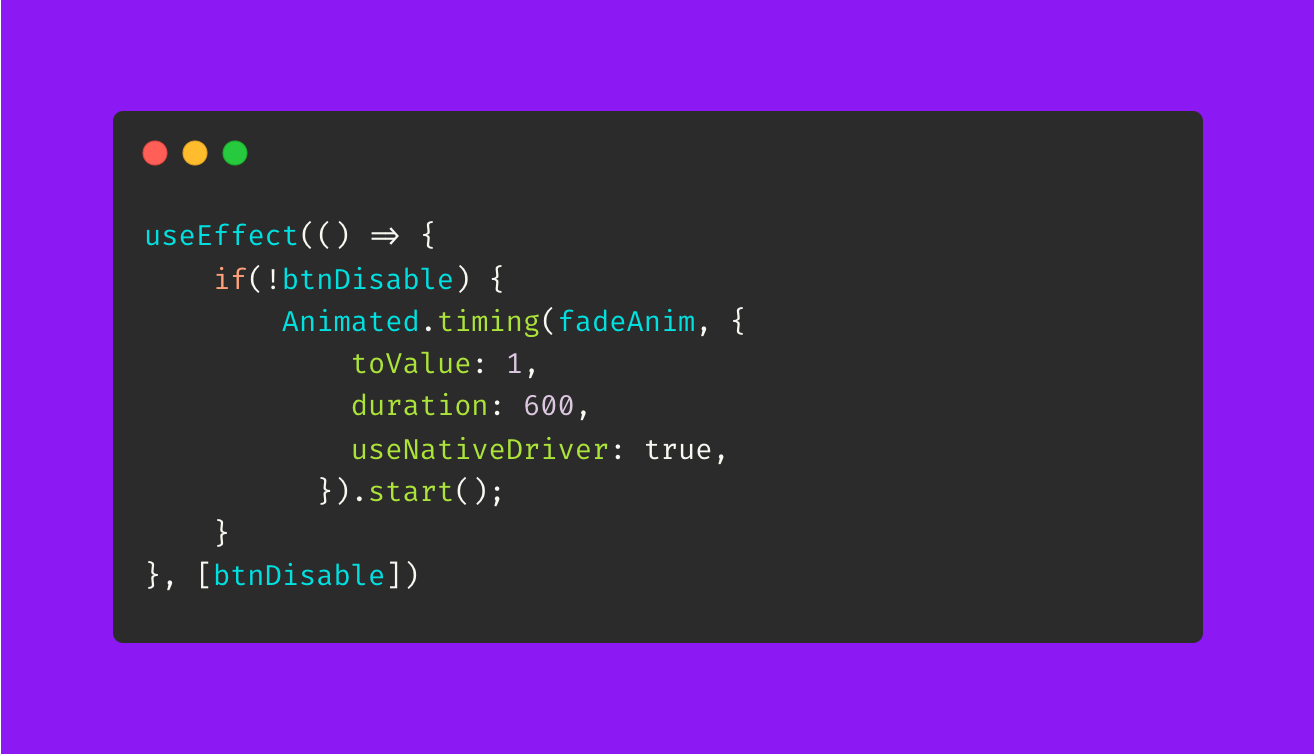
and this is our button component.

3. Tracking and showing the progress bar
Our last task is to track the progress of the user’s scroll against the full height of the page. In order to track the progress, we will use an animated value in our onScroll function.
const AnimatedProgressValue = new Animated.Value(0)
The following code describes the process of tracking the progress.
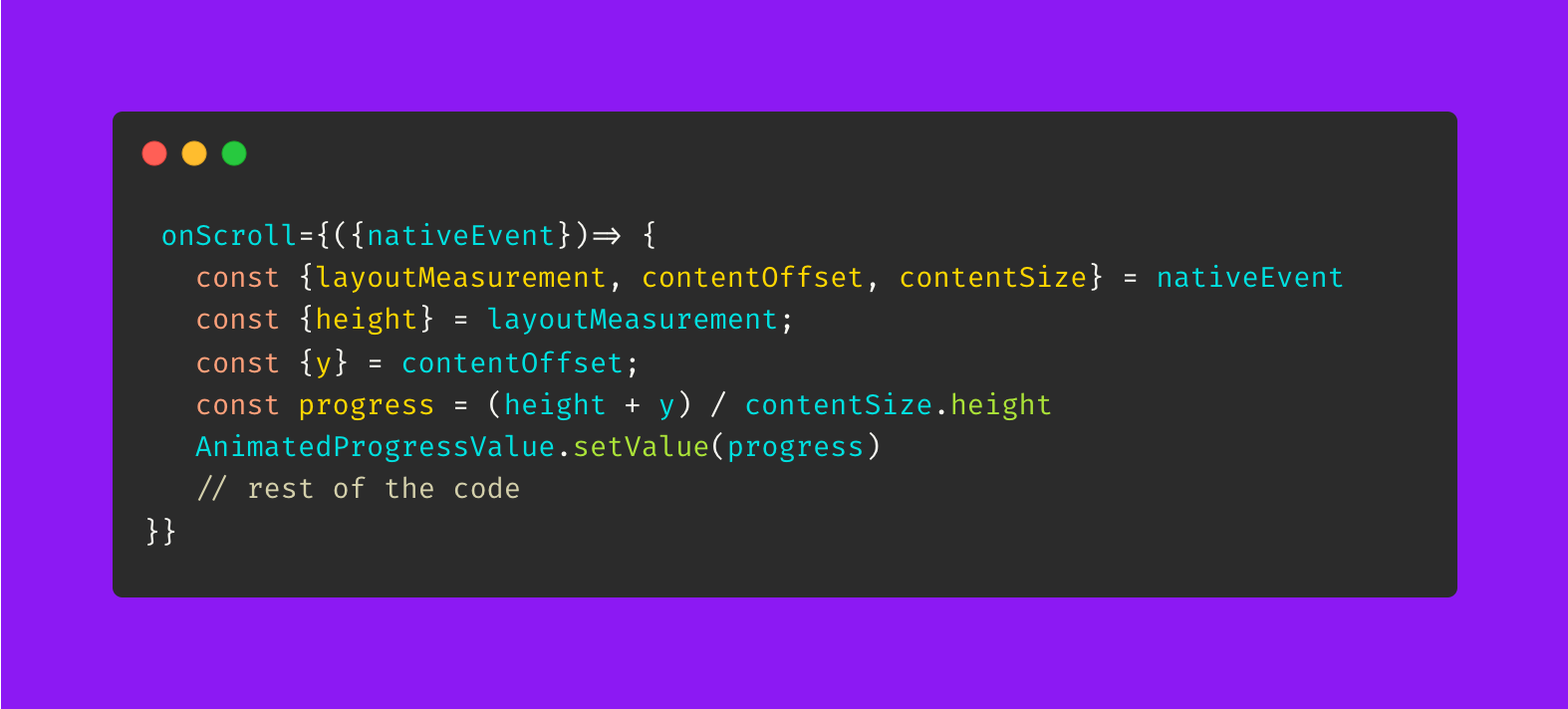
We have the progress value in our AnimatedProgressValue
variable. We can use this to generate the progress bar at the bottom of the page. This implementation is the following
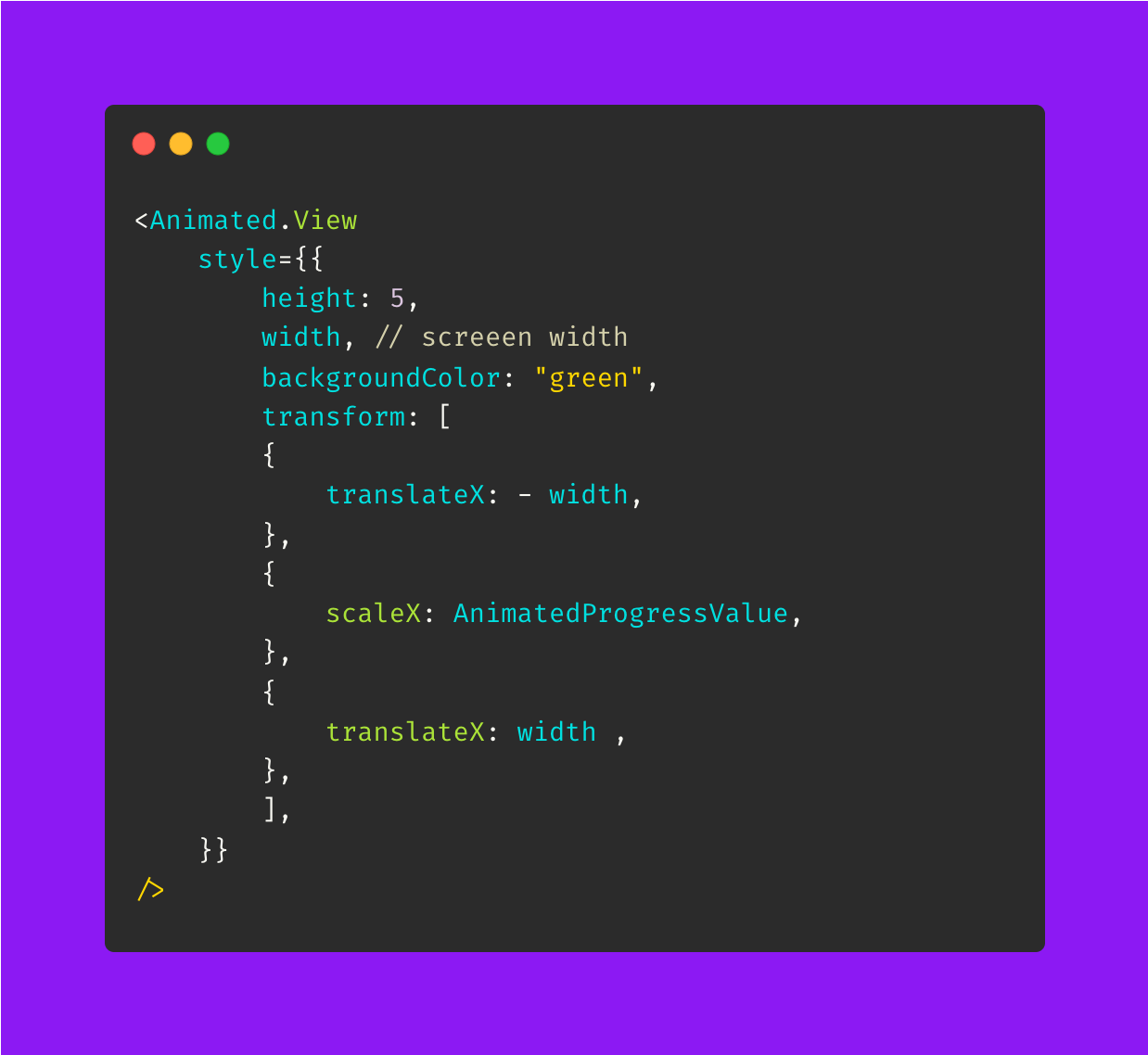
The progress bar has a width equal to the width of the screen. We just need to increase the scaleX
according to progress value. The translateX
is needed to scale from the left, otherwise, the scale starts to happen from the center, It works like transformOrigin: '0px 0px'
the web.
Here is the full code and demo of the post – https://snack.expo.dev/@saad-bashar/terms-conditions-demo